C Programming Full Course –
Introduction to C Programming
- Overview:
- Explore the fundamentals of the C language.
- Understand its historical significance and enduring popularity.
- Structure of a C Program:
- Dive into the essential components that make up a C program.
🌟 Basics of C Programming
- Data Types and Variables:
- Learn about various data types and how to declare variables.
- Input and Output Functions:
- Master the printf and scanf functions for effective communication with the user.
- Arithmetic Operations:
- Perform basic mathematical operations and enhance your numerical skills.
🛠️ Control Flow
- Decision-Making Statements:
- Utilize if, else, and switch statements for effective decision-making.
- Looping Statements:
- Master for, while, and do-while loops for efficient repetition.
📋 Functions
- Function Definition and Declaration:
- Understand the anatomy of functions and how to declare and define them.
- Function Parameters and Return Values:
- Explore the power of parameters and return values in functions.
- Recursion:
- Dive into the concept of recursive functions for elegant problem-solving.
📊 Arrays
- Declaration and Initialization:
- Learn how to declare and initialize arrays for efficient data storage.
- Array Manipulation and Operations:
- Master array manipulation techniques for effective data handling.
🔗 Pointers
- Understanding Pointers:
- Unlock the mysteries of pointers and their role in memory manipulation.
- Pointer Arithmetic:
- Dive into advanced pointer concepts, including arithmetic operations.
- Dynamic Memory Allocation:
- Explore dynamic memory allocation with
malloc
andfree
.
- Explore dynamic memory allocation with
🧵 Strings
- String Manipulation:
- Learn various string manipulation techniques.
- String Input and Output:
- Master handling strings with input and output operations.
- String Handling Functions:
- Explore built-in functions for efficient string manipulation.
🏗️ Structures and Unions
- Defining Structures:
- Create complex data structures using structures.
- Nesting Structures:
- Learn the art of nesting structures for more sophisticated data organization.
- Union Concept:
- Explore the concept of unions for efficient memory usage.
📁 File Handling
- Opening, Closing, and Reading Files:
- Master file handling operations for input and output.
- Writing Data to Files:
- Learn how to write data to files for persistent storage.
- Random Access in Files:
- Explore random access techniques for efficient data retrieval.
🚀 Advanced Concepts
- Dynamic Memory Allocation and Deallocation:
- Understand advanced memory management techniques.
- Preprocessor Directives:
- Dive into preprocessor directives for code customization.
- Bitwise Operations:
- Unlock the power of bitwise operations for efficient coding.
- Typedef and Enum:
- Utilize
typedef
andenum
for enhanced code readability.
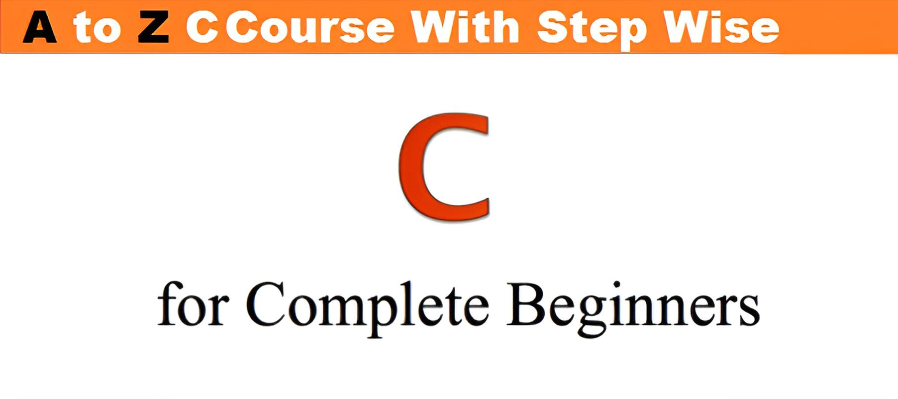
🚨 Error Handling
- Understanding Errors and Exceptions:
- Learn how to identify, handle, and recover from errors.
- Error Handling Techniques:
- Explore various error-handling techniques for robust code.
🌐 Data Structures in C
- Linked Lists:
- Dive into the world of linked lists for dynamic data storage.
- Stacks and Queues:
- Explore stack and queue data structures and their applications.
- Trees and Graphs:
- Understand hierarchical data structures like trees and graphs.
📚 C Standard Library
- Overview of Standard Library Functions:
- Explore commonly used functions from
stdio.h
,stdlib.h
,string.h
, etc.
- Explore commonly used functions from
- Utilizing Standard Library Functions:
- Master the art of leveraging standard library functions for efficient coding.
🌐 C Programming Best Practices
- Code Organization and Documentation:
- Develop good coding habits for maintainable and scalable projects.
- Debugging Techniques:
- Learn effective debugging strategies for quick issue resolution.
- Coding Standards:
- Understand the importance of coding standards for collaborative development.
🏗️ Building Larger Projects
- Modular Programming:
- Organize code into modular components for scalability.
- Header Files and Source Files:
- Master the use of header files and source files for code separation.
- Makefiles:
- Learn how to create Makefiles for efficient project compilation.
🔄 Introduction to Algorithms
- Sorting and Searching Algorithms:
- Dive into essential algorithms for sorting and searching.
- Time and Space Complexity Analysis:
- Understand the efficiency of algorithms through time and space complexity analysis.
🏆 Practice and Projects
- Solving Coding Exercises:
- Sharpen your skills with a variety of coding exercises.
- Building Small Projects:
- Apply your knowledge by working on small projects for hands-on experience.
📚 Learning Resources
- Recommended Books, Tutorials, and Online Courses:
- Explore curated resources to deepen your understanding.
- Practice Platforms and Coding Challenges:
- Engage with coding challenges on various platforms to enhance your skills.
See Also – Hello World program step by step execution
Latest posts by Mahesh Wabale (see all)
- Cursor AI: Why use Cursor AI? - July 9, 2025
- Grafana Setup - June 30, 2025
- What is Grafana? - June 30, 2025