Introduction to printf and scanf in C
Introduction:
In the realm of programming, understanding the basic principles of input and output (I/O) is fundamental, and this assignment introduces these concepts using the C programming language. Specifically, the focus is on the functions printf and scanf, which are crucial for displaying information to the user and obtaining input from them.
See Also – Loops in C
Description:
The program begins with the inclusion of the stdio.h library, a standard library in C that provides functions for handling input and output operations. The main function is the starting point of the program’s execution. The initial action involves using the printf function to display the message “Hello, World!” on the console. This is a customary practice in programming to initiate learners to the syntax and structure of a basic program.
Following the display of the message, the program moves on to the next step, which is prompting the user to input a number. The scanf function is employed for this purpose, and it waits for the user to provide an integer. The %d in the scanf function is a format specifier, indicating that the input should be interpreted as an integer.
After capturing the user’s input, the program utilizes printf once again to echo back the entered number to the user. This part of the program demonstrates the bidirectional nature of I/O operations, where the program communicates with the user by both displaying information and receiving input.
Theory:
The #include <stdio.h> directive is crucial for incorporating the standard input-output library, providing the necessary functions for I/O operations. The main function is the entry point of any C program, and within it, the printf function is used for formatted output, while scanf is employed for formatted input. The %d in scanf and printf is a format specifier, representing that the associated variable is of integer type.
Program:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
int num;
printf("Enter a number: ");
scanf("%d", &num);
printf("You entered: %d\n",num);
return 0;
}
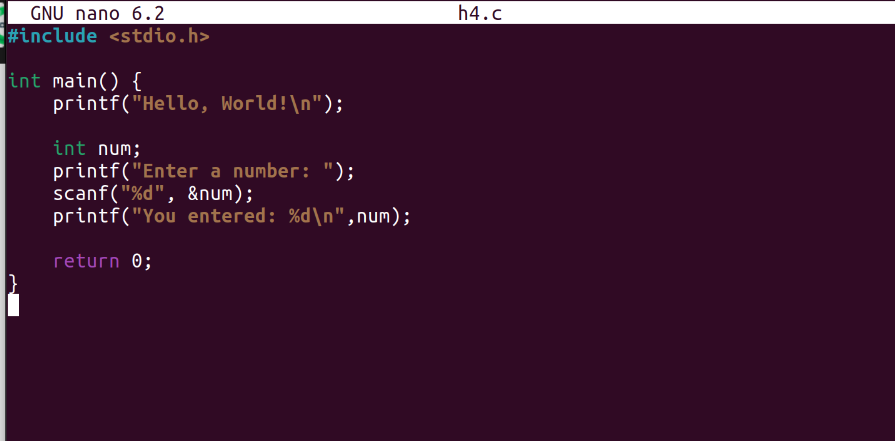
Output:
Hello, World!
Enter a number: 42
You entered: 42
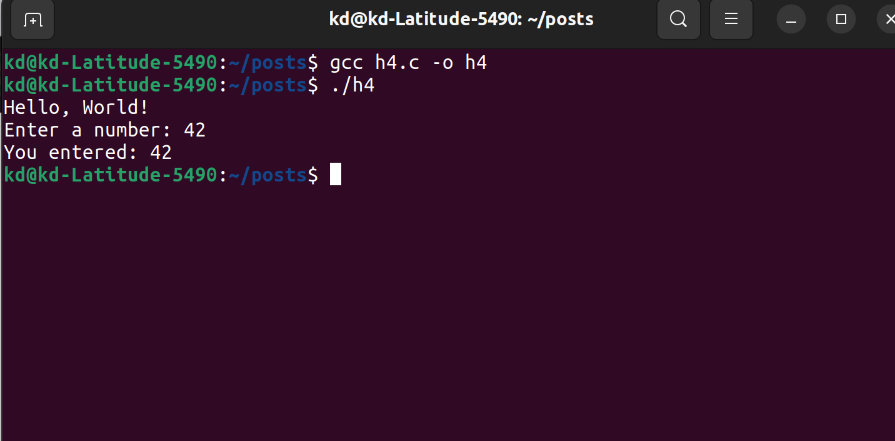
- Deployment of Spring Boot Application on Kubernetes Using Helm - July 19, 2024
- Robot Framework for Selenium Automation - July 14, 2024
- Pytest Framework (Selenium Automation) - July 14, 2024