C language Toolchain : Step-by-step guide for the execution of the C language toolchain.
Step 1: Install GCC
C language Toolchain : If you don’t have GCC installed, you can install it using the package manager:
$ sudo apt-get update $ sudo apt-get install gcc $ sudo apt-get install build-essential
The steps of execution of C program are C code then Preprocessing then Compiler then Assembler then Linker then Loader. In Preprocessing source code is attached to the preprocessor file. Different types of header files are used like the studio. h, math.
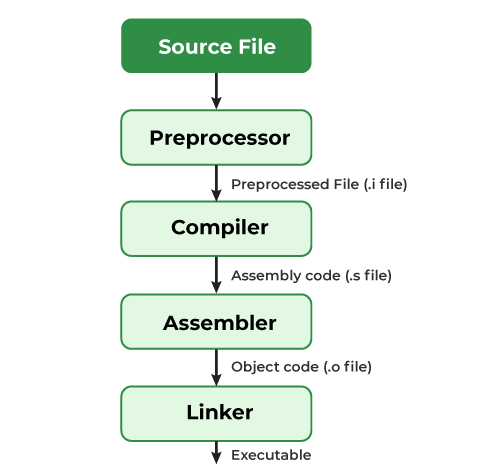
Let’s break down the entire process of compiling and executing a simple “Hello, World!” program in C. The steps involve the preprocessor, compiler, assembler, linker, and finally, the loader for execution.

Overview of the steps involved in the execution of a C program, covering the roles of the editor, preprocessor, compiler, assembler, linker, and RAM:
- Editor:
- Role: The editor is a text editor where the programmer writes the C code. Examples include Notepad, Vim, Emacs, or modern integrated development environments (IDEs) like Visual Studio Code, Eclipse…etc
- Function: It provides a user interface for writing, modifying, and saving the C source code.
- Preprocessor:
- Role: The preprocessor is a program that processes the C source code before actual compilation.
- Function:
- It handles directives starting with
#
(such as#include
,#define
) and performs text substitution. - It includes header files and expands macros.
- It removes comments.
- It prepares the code for compilation.
- It handles directives starting with
- Compiler:
- Role: The compiler translates the preprocessed C code into assembly code or an intermediate representation.
- Function:
- It checks the syntax and semantics of the code.
- It generates assembly code or an intermediate form.
- It may perform optimizations to improve code efficiency.
- Assembler:
- Role: The assembler converts the assembly code into machine code or object code.
- Function:
- It translates human-readable assembly code into binary machine code.
- It handles symbols and addresses, producing an object file.
- Linker:
- Role: The linker combines multiple object files (generated by the compiler and assembler) into a single executable program.
- Function:
- It resolves addresses between different object files.
- It links library functions.
- It produces the final executable file.
- RAM (Random Access Memory):
- Role: RAM is a type of computer memory that is used to store data and instructions temporarily during program execution.
- Function:
- The compiled program, along with necessary data, is loaded into RAM during execution.
- RAM is volatile memory, meaning it loses its contents when the power is turned off.
- The CPU reads and executes instructions from RAM, providing fast access to data during runtime.
See Also – Data Types in C
Assuming you have a file named `hello.c` with the “Hello, World!” program:
// hello.c #include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
Here’s the step-by-step process:
1. Preprocessor:
The preprocessor is responsible for handling directives and macro substitutions.
$ gcc -E hello.c -o hello.i
This generates the preprocessed file `hello.i`.
2. Compiler:
The compiler translates the preprocessed code into assembly code.
$ gcc -S hello.i -o hello.s
This produces the assembly code file `hello.s`.
3. Assembler:
The assembler converts the assembly code into machine code, producing an object file.
$ gcc -c hello.s -o hello.o
This generates the object file `hello.o`.
4. Linker:
The linker combines object files and libraries to create an executable.
$ gcc hello.o -o hello
This produces the executable file `hello`.
5. Loader and Execution:
The loader loads the executable into memory, and the program is executed.
$ ./hello

This executes the “Hello, World!” program and displays the output.
Putting It All Together:
$ gcc -E hello.c -o hello.i # Preprocessing $ gcc -S hello.i -o hello.s # Compilation to Assembly $ gcc -c hello.s -o hello.o # Assembly to Object $ gcc hello.o -o hello # Linking $ ./hello # Execution
After running these commands, you should see the output:
Hello, World!
This sequence represents the complete compilation and execution flow of a simple C program.
Step 6: Examine Assembly Code (Optional):
If you want to inspect the generated assembly code, you can use the cat command:
$ cat hello.s
This command displays the content of the assembly file.
Step 7: Debugging with GDB (Optional):
If you want to debug the program using GDB, you can use the following commands:
$ gdb ./hello
Inside GDB, you can use commands like run, break main, and continue to navigate and debug the program.
That’s it! You’ve successfully executed the C language toolchain on Ubuntu using GCC.
- Grafana Setup - June 30, 2025
- What is Grafana? - June 30, 2025
- Deploy Apache Tomcat Using Ansible - June 30, 2025