Arrays in C Programming –
1. Introduction to Arrays
Arrays are a collection of elements of the same data type stored in contiguous memory locations. They provide a convenient way to organize and access multiple values under a single identifier.
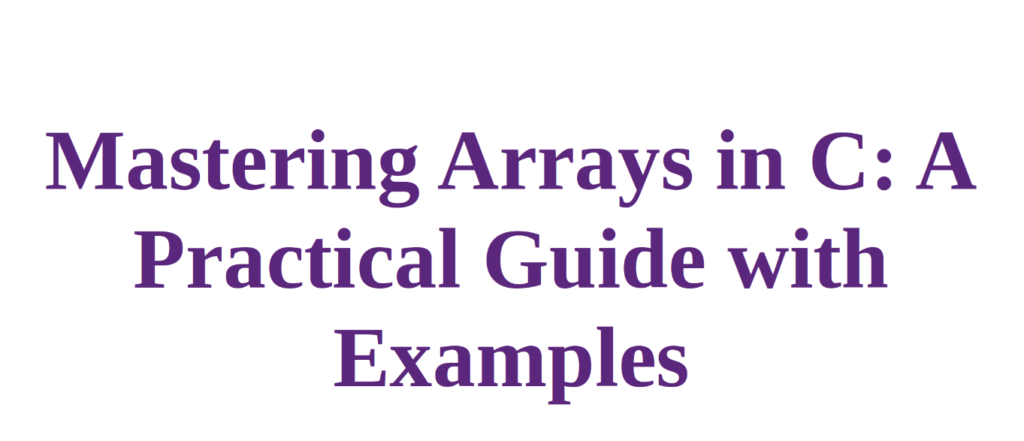
Declaration and Initialization:
In C, you declare an array using the syntax:
dataType arrayName[arraySize];
Here, dataType
is the type of elements, arrayName
is the identifier, and arraySize
represents the number of elements.
See Also – Introduction to printf and scanf in C
int numbers[5]; // Declaration of an integer array with size 5
2. Array Indexing and Accessing Elements
Arrays use zero-based indexing, meaning the first element is at index 0.
Zero-based Indexing:
int firstElement = numbers[0];
Accessing Elements using Subscripts:
You can access and modify elements using square brackets and the index:
numbers[2] = 42; // Modifying the third element
3. Multi-dimensional Arrays
Multi-dimensional arrays allow you to store data in multiple dimensions, such as rows and columns.
Two-dimensional Arrays:
int matrix[3][3]; // Declaration of a 3x3 integer matrix
matrix[1][2] = 10; // Accessing and modifying an element
Nested Loops:
Iterating through a two-dimensional array using nested loops:
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
// Accessing matrix[i][j]
}
}
4. Array Operations and Manipulations
Traversing Arrays:
Loop through an array to access and process each element:
for (int i = 0; i < 5; ++i) {
// Accessing numbers[i]
}
Modifying Array Elements:
for (int i = 0; i < 5; ++i) {
numbers[i] *= 2; // Doubling each element
}
Copying and Concatenating Arrays:
int copy[5];
for (int i = 0; i < 5; ++i) {
copy[i] = numbers[i]; // Copying elements
}
5. String Handling with Arrays
C-style strings are character arrays terminated by a null character ('\0'
).
C-Style Strings:
char greeting[6] = "Hello";
String Manipulation Functions:
Using library functions like strcpy
, strcat
, and strlen
:
char destination[12];
char source[] = " World!";
strcpy(destination, greeting); // Copying strings
strcat(destination, source); // Concatenating strings
Common String Operations:
int length = strlen(greeting); // Finding the length
6. Dynamic Memory Allocation for Arrays
Dynamic memory allocation allows you to create arrays with a size determined at runtime.
Using malloc
, calloc
, and realloc
:
int *dynamicArray = (int *)malloc(5 * sizeof(int));
Memory Deallocation with free
:
free(dynamicArray);
Handling Memory Allocation Errors:
Always check if dynamic memory allocation is successful:
if (dynamicArray == NULL) {
// Handle allocation failure
}
7. Passing Arrays to Functions
Arrays are typically passed to functions by reference, allowing modifications to affect the original array.
Passing Arrays to Functions by Reference:
void modifyArray(int arr[], int size) {
for (int i = 0; i < size; ++i) {
arr[i] += 5; // Modifying array elements
}
}
Array Size and Function Parameters:
void printArray(int arr[], int size) {
for (int i = 0; i < size; ++i) {
printf("%d ", arr[i]); // Printing array elements
}
printf("\n");
}
Function Return Values with Arrays:
int *createArray(int size) {
int *newArray = (int *)malloc(size * sizeof(int));
// Initialize and populate the array
return newArray;
}
8. Sorting and Searching Algorithms
Implementing common sorting and searching algorithms with arrays.
Bubble Sort, Insertion Sort, and Selection Sort:
void bubbleSort(int arr[], int size);
void insertionSort(int arr[], int size);
void selectionSort(int arr[], int size);
Binary Search and Linear Search:
int binarySearch(int arr[], int size, int target);
int linearSearch(int arr[], int size, int target);
Performance Considerations and Trade-offs:
Choosing the right algorithm based on the size and nature of the data.
9. Arrays and Pointers
Arrays and pointers are closely related concepts in C.
Relationship between Arrays and Pointers:
int arr[5];
int *ptr = arr; // Pointer pointing to the first element
Pointer Arithmetic and Array Manipulation:
int thirdElement = *(ptr + 2); // Accessing the third element using pointer arithmetic
Dynamic Arrays using Pointers:
int *dynamicArr = (int *)malloc(5 * sizeof(int));
10. Best Practices and Tips
Guidelines for efficient and safe array usage.
Memory Management and Avoiding Memory Leaks:
free(dynamicArr); // Freeing dynamically allocated memory
11. Example
Let’s simplify it while still covering the essential concepts. Below is a more concise program that includes array declaration, initialization, manipulation, and dynamic memory allocation:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Function prototypes
void printArray(int arr[], int size);
void modifyArray(int arr[], int size);
int main() {
// Introduction to Arrays
int numbers[5] = {10, 5, 8, 2, 7};
// Array Indexing and Accessing Elements
int firstElement = numbers[0];
numbers[2] = 42;
// Array Operations and Manipulations
printArray(numbers, 5);
modifyArray(numbers, 5);
printArray(numbers, 5);
// String Handling with Arrays
char greeting[6] = "Hello";
char destination[12];
strcpy(destination, greeting);
printf("Concatenated String: %s\n", destination);
// Dynamic Memory Allocation for Arrays
int *dynamicArray = (int *)malloc(5 * sizeof(int));
if (dynamicArray == NULL) {
fprintf(stderr, "Memory allocation failed.\n");
return 1;
}
for (int i = 0; i < 5; ++i) {
dynamicArray[i] = i * 2;
}
printArray(dynamicArray, 5);
free(dynamicArray);
return 0;
}
// Function definitions
void printArray(int arr[], int size) {
printf("Array: ");
for (int i = 0; i < size; ++i) {
printf("%d ", arr[i]);
}
printf("\n");
}
void modifyArray(int arr[], int size) {
for (int i = 0; i < size; ++i) {
arr[i] += 5;
}
}
This program covers a range of array-related concepts and provides practical examples.
- AnchorSetup using Docker-Compose - October 18, 2024
- Devops assignment - October 17, 2024
- Deployment of vault HA using MySQL - September 18, 2024